ICSE Java Programming Electricity Bill 2017 Q4 Solved
Question 4.
Define a class Electricity Bill with the following specifications : [15]
class : ElectricBill
Instance variables /data member :
String n – to store the name of the customer
int units – to store the number of units consumed
double bill – to store the amount to be paid
Member methods :
void accept ( ) – to accept the name of the customer and number of units consumed
void calculate ( ) – to calculate the bill as per the following tariff :
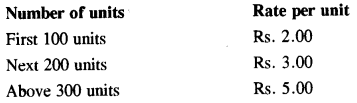
A surcharge of 2.5% charged if the number of units consumed is above 300 units.

Write a main method to create an object of the class and call the above member methods.
Python Code
class ElectricityBill:
def accept(self):
self.customer_Name = str(input("Enter the name of the customer:"))
self.units_Consumed = int(input("Enter the units consumed:"))
def calculate(self):
if self.units_Consumed <= 100:
self.bill_Amount = self.units_Consumed *2.0
elif self.units_Consumed > 100 and self.units_Consumed <= 300:
self.bill_Amount = self.units_Consumed *3.0
else:
self.bill_Amount = self.units_Consumed *5.0
self.bill_Amount += self.bill_Amount*(2.5/100.0)
def printBill(self):
print("Name of the customer: ",self.customer_Name)
print("Total units consumed: ",self.units_Consumed)
print("Bill Amount: ",self.bill_Amount)
def __init__(self) -> None:
self.customer_Name = ""
self.units_Consumed = 0
self.bill_Amount =0.0
obj = ElectricityBill()
obj.accept()
obj.calculate()
obj.printBill()
JAVA Code
import java.util.Scanner;
public class ICSEJava {
public static void main(String[] args) {
ElectricityBill obj = new ElectricityBill();
obj.accept();
obj.calculate();
obj.print();
}
}
class ElectricityBill {
public void print() {
System.out.println("Name of the customer: " + this.customer_Name);
System.out.println("Total units consumed: " + this.units_Consumed);
System.out.println("Bill Amount: " + this.bill_Amount);
}
public void calculate() {
if (this.units_Consumed <= 100)
this.bill_Amount = this.units_Consumed * 2.0;
else if (this.units_Consumed > 100 && this.units_Consumed <= 300)
this.bill_Amount = this.units_Consumed * 3.0;
else {
this.bill_Amount = this.units_Consumed * 5.0;
this.bill_Amount += (this.bill_Amount)*(2.5/100);
}
}
public void accept() {
Scanner in = new Scanner(System.in);
System.out.println("Enter the name of the customer.");
this.customer_Name = in.nextLine();
System.out.println("Enter the unit consumed.");
this.units_Consumed = in.nextInt();
in.close();
}
ElectricityBill() {
this.bill_Amount = 0.0;
this.units_Consumed = 0;
this.customer_Name = "";
}
private int units_Consumed;
private String customer_Name;
private double bill_Amount;
}
No comments:
Post a Comment